APIs & Features
After you have successfully completed the steps from the
previous section you are now ready to use an instance of CardsService
to work with your customers card details.
Listening to events
You can use the following code in order to listen to events emitted by Cards SDK
|
|
To check SDK setup success
Use the following piece of code to check if your SDK was set up successfully.
|
|
To log your users out
Use the following code to log your current users out
|
|
Features
This section covers the functionalities that the Cards SDK has on offer
Get card view UI
Cards SDK provides the card view which you can use directly in your app. You can get the card view with the layout and styles configured specifically for your app with the Zeta platform. The default state of the view returned is the collapsed view which shows only the card PAN.
On clicking the view, the user can toggle between the collapsed and the sensitive info states. User authentication is required for accessing the sensitive state of the card’s view if the user has not been asked for authentication already in the current app session.
|
|
Properties supported
- cardId - (String) Compulsory property. This denotes the card ID for which the card view is required. For example: CARD_ID = “123456789”
- templateId - (String) Optional property. This denotes the template ID you want to render. For example: TEMPLATE_ID = “card_EN_UIVIEW”.
- jsonPayload - (Object) Optional property. This denotes the additional payload you want to send. For example: JSON_PAYLOAD = { name: ‘user_name’, company: ‘zeta’ }.
Sample Screens
Collapsed view
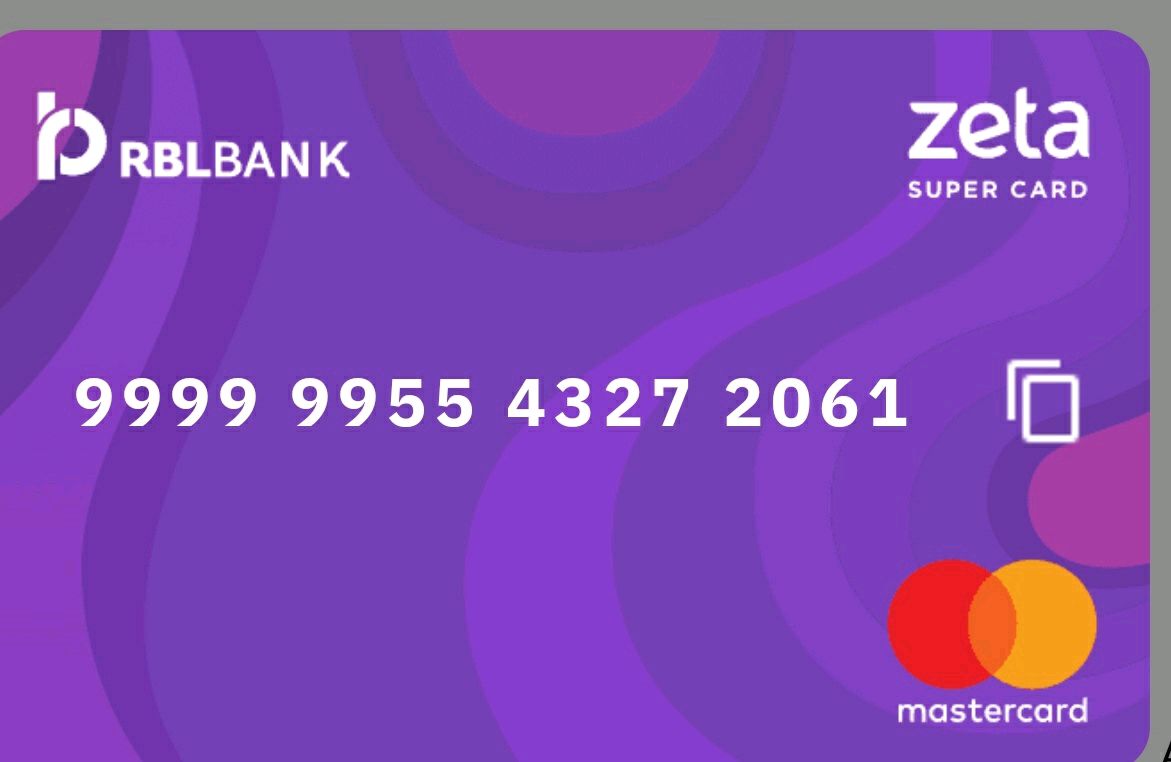
Sensitive data view
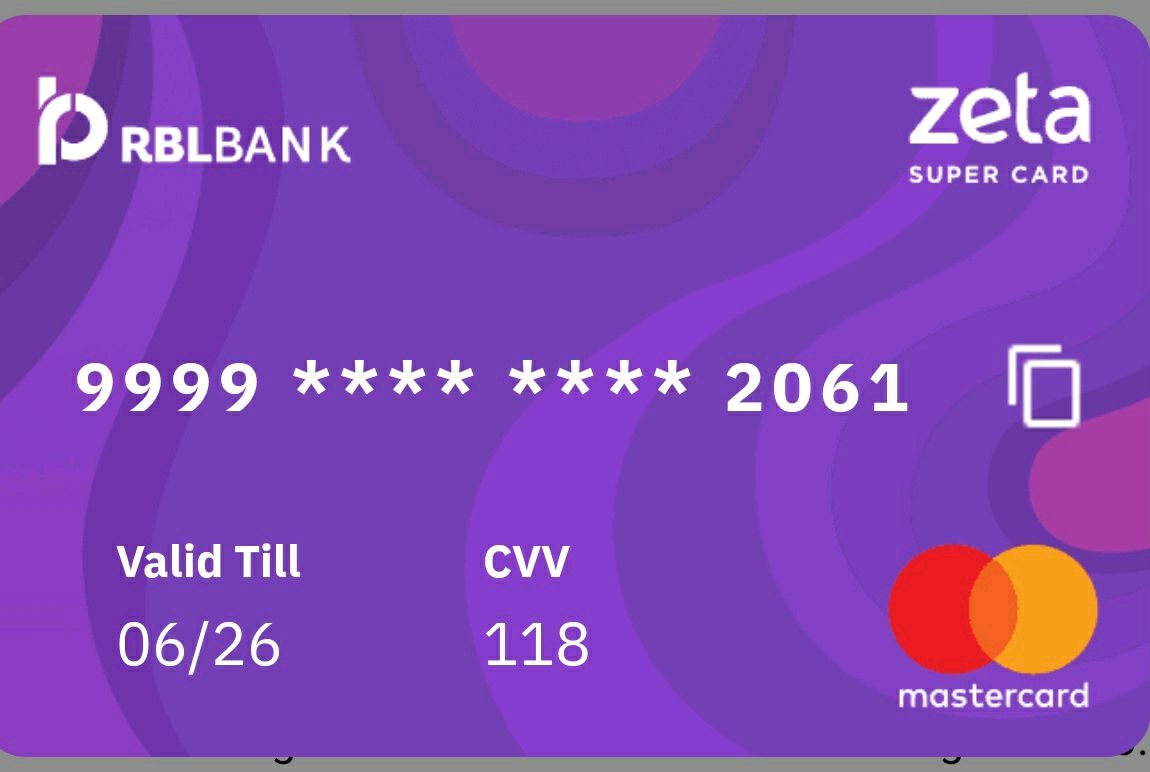
Copy Card number
Card SDK provides the functionality to copy the un-masked card number. On success, it shows a toast message with the card number. Post that, you can paste the card number where-ever required.
Here is the code snippet for the same.
|
|
Set Static PIN UI
Cards SDK also provides a UI flow for setting the Static PIN. The UI is in accordance with the theme with which the SDK has been configured. The UI allows the user to enter the Static PIN twice. The entered PIN has validations for PIN length and equality. Upon authentication, the PIN is stored in an encrypted store.
Here is the code snippet for the same.
|
|
Sample response
- Set Static PIN UI - Initial
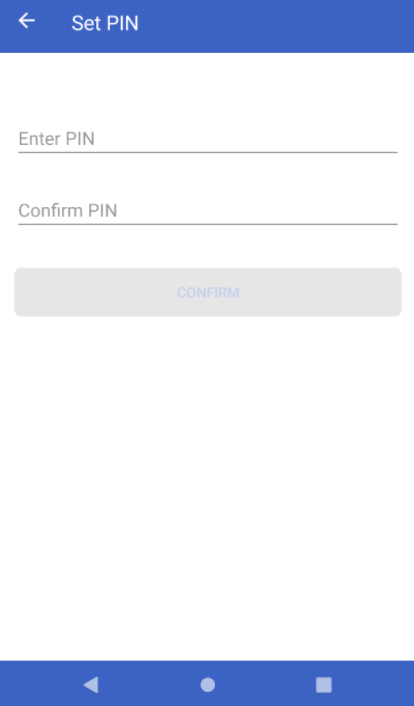
- Set Static PIN UI - Incorrect PIN
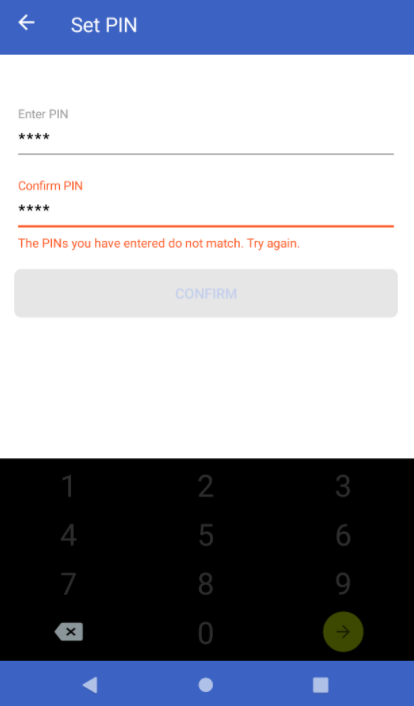
- Set Static PIN UI - Correct PIN
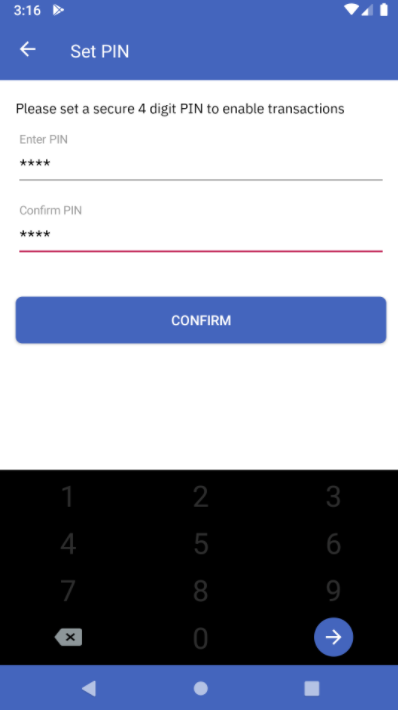
- Set Static PIN UI - Success
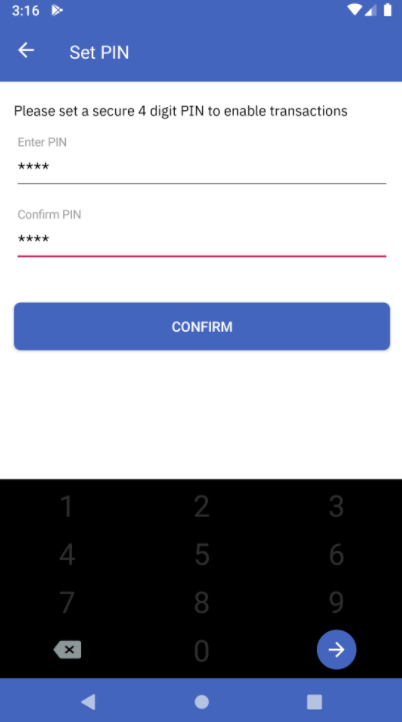
Change Static PIN UI
Cards SDK also provides a UI for changing the Static PIN given a card form-factor. The UI is in accordance with the theme with which the SDK has been configured. This feature sits behind user-authentication and requires authentication per launch of the app. The UI comprises of three input fields where we can enter:
- Old Static PIN
- New Static PIN
- Re-enter New Static PIN
Users can update the Static by providing the correct Old PIN and valid new PIN. The UI also provides validations for:
- PIN lengths
- Equality of the New Static PIN entered twice.
Here is the code snippet for the same.
|
|
Sample response
- Change Static PIN UI - Initial
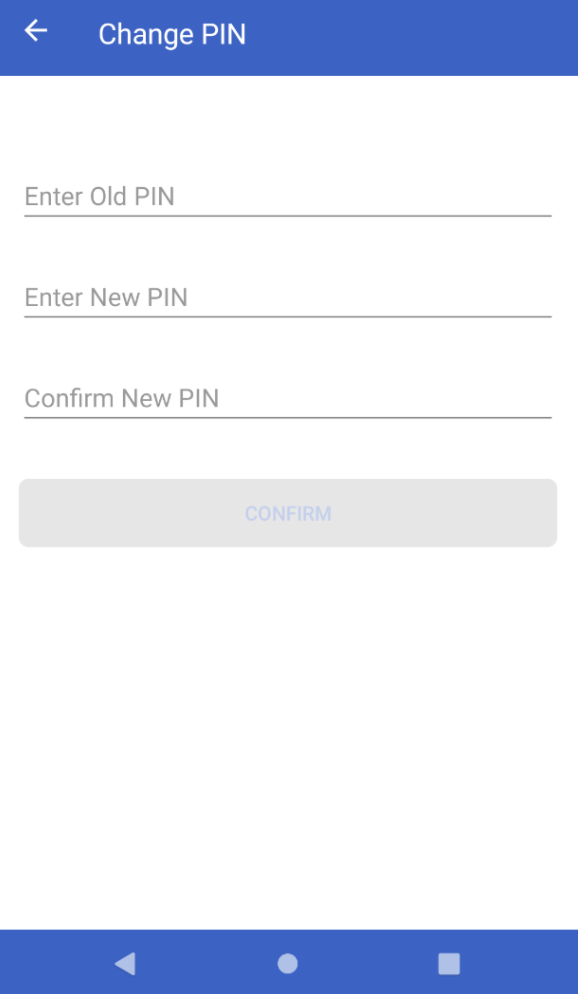
- Change Static PIN UI - New PIN Mismatch
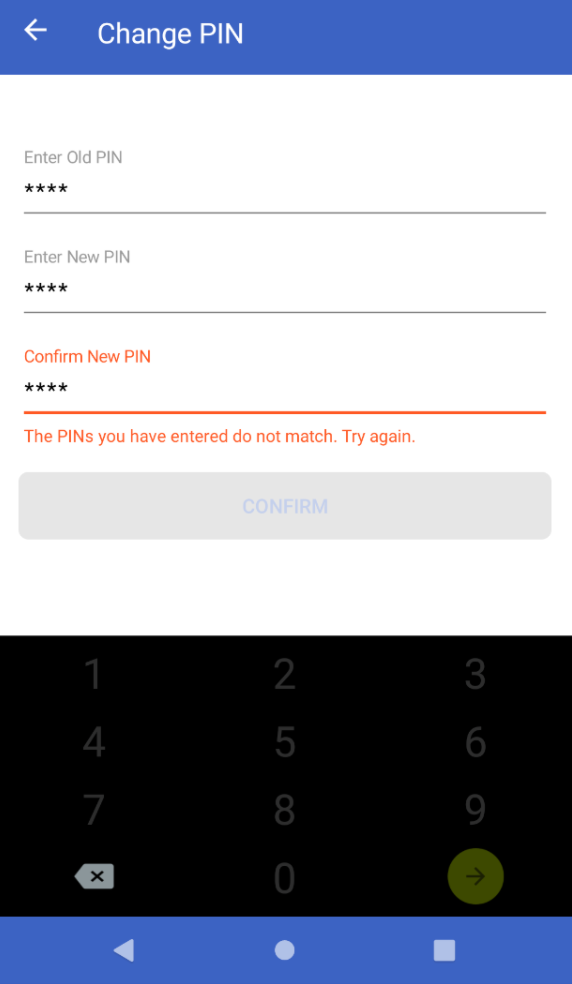
- Change Static PIN UI - Success
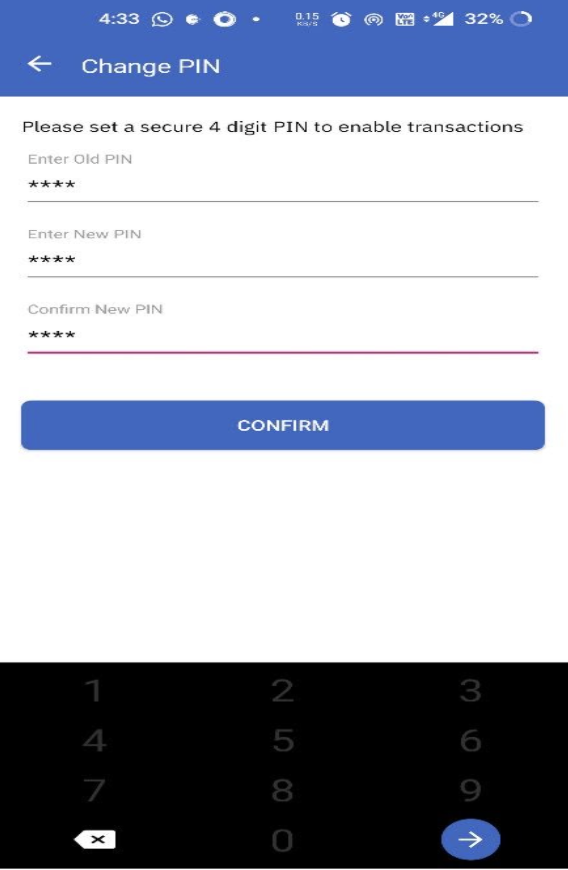
Super PIN
Super PIN is a time-synced based dynamic pin that changes every 2 minutes (configurable). The app can query for the Super PIN for different resources associated with the user.
Get Super PIN UI
Note: Use this only when you are sure that users have a single resource associated with their accountHolderId’s
Cards SDK also provides a UI for fetching the Super PIN. The UI adds a translucent black background on the existing screen and shows a popup containing the Super PIN. The UI is in accordance with the theme with which the SDK has been configured.
Here is a code snippet on how you can fetch the Super PIN UI assuming a single resource
|
|
Sample response
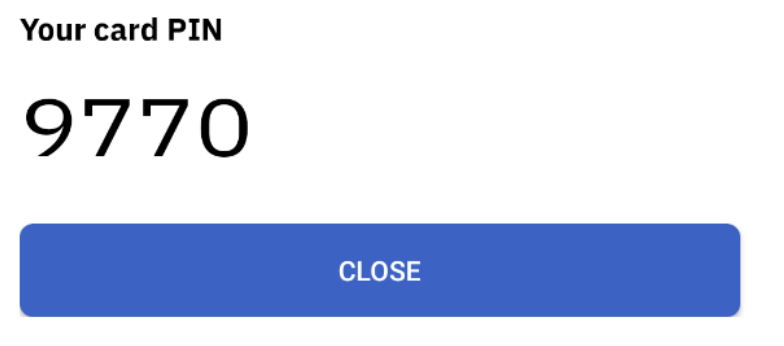
Get Super PIN API
Note: Use this only when you are sure that users have a single resource associated with their accountHolderId’s
The return value is a Super PIN along with the validity in milliseconds.
Here is a code snippet on how you can fetch the Super PIN assuming a single resource.
|
|
Get super PIN for resource UI
Cards SDK also provides a UI for fetching the Super PIN for a given resource. The UI adds a translucent black background on the existing screen and shows a popup containing the Super PIN. The UI is in accordance with the theme with which the SDK has been configured.
Here is a code snippet on how you can fetch the Super PIN UI for a resource.
|
|
Sample response
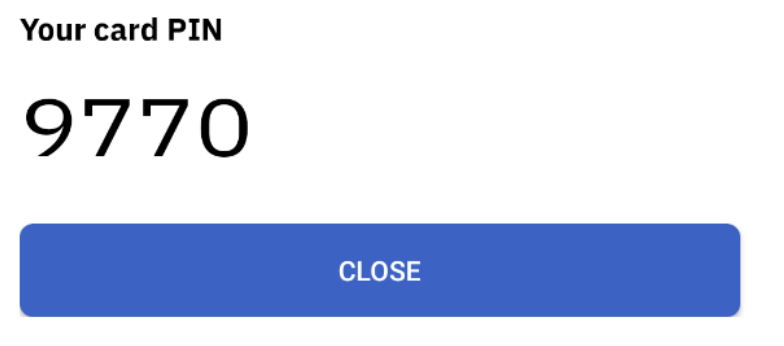
Get super PIN for resource API
The return value is a Super PIN along with the validity in milliseconds.
Here is a code snippet on how you can fetch the Super PIN for a given resource.
|
|
Block Card UI
Cards SDK also provides a UI for blocking the card. The UI is in accordance with the theme with which the SDK has been configured. The UI adds a translucent black background on the existing screen and shows a snackbar containing the option to either cancel or Block the card.
Here is the code snippet for the same.
|
|
Sample response
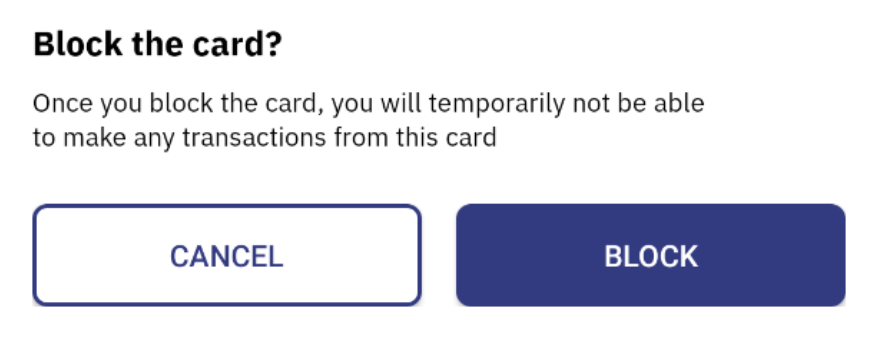
Block Card API
Cards SDK exposes this API to expose the Block Card functionality. The user can use this API to block the card temporarily. The user may unblock the card in future if required.
Here is the code snippet for the same.
|
|
Unblock Card UI
Cards SDK also provides a UI for unblocking the card. The UI is in accordance with the theme with which the SDK has been configured. The UI adds a translucent black background on the existing screen and shows a snackbar containing the option to either cancel or Unblock the card.
Here is the code snippet for the same.
|
|
Sample response
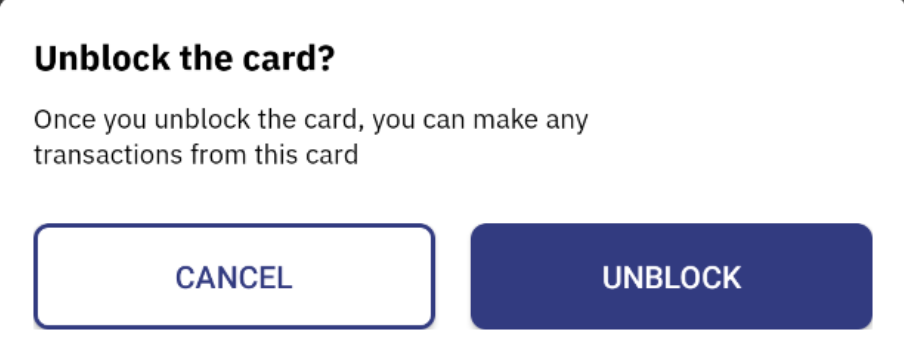
Unblock Card API
Cards SDK exposes this API to expose the Unblock Card functionality. The user can use this API to unblock the card which was blocked temporarily using the Block Card API.
Here is the code snippet for the same.
|
|
Front Face Card View UI
Cards SDK provides a full equipped front face card UI. This is a full UI for all the features provided by SDK cards. This UI supports multiple customisations and can be completely designed and changed from as per client requirement.
Here is a code snippet.
|
|
Here are some of the UI designs supported with Front Face Card UI:
Design 1
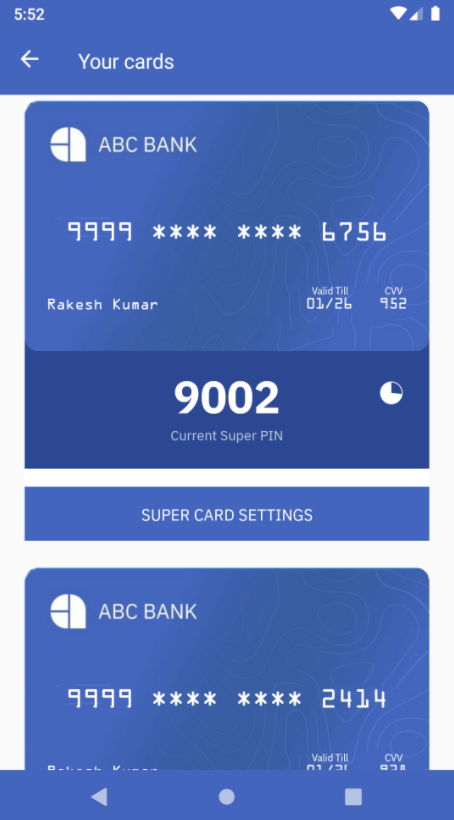
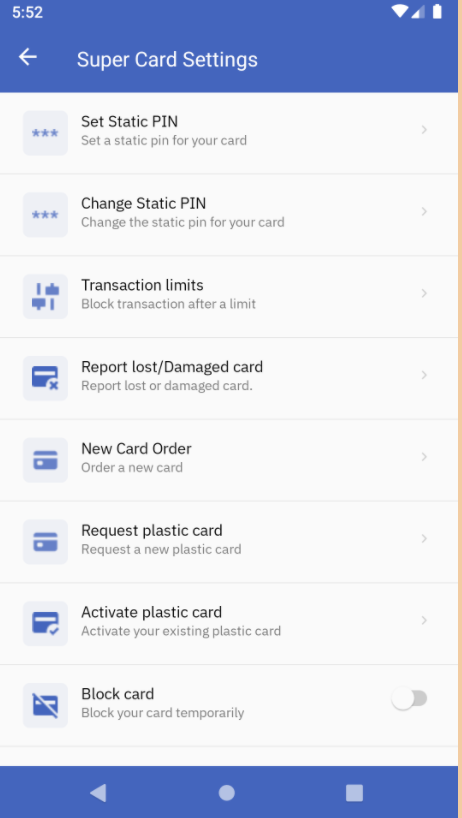
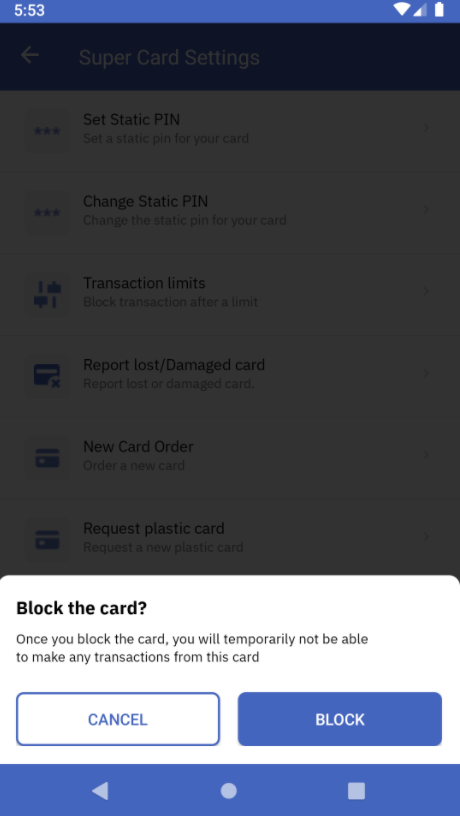
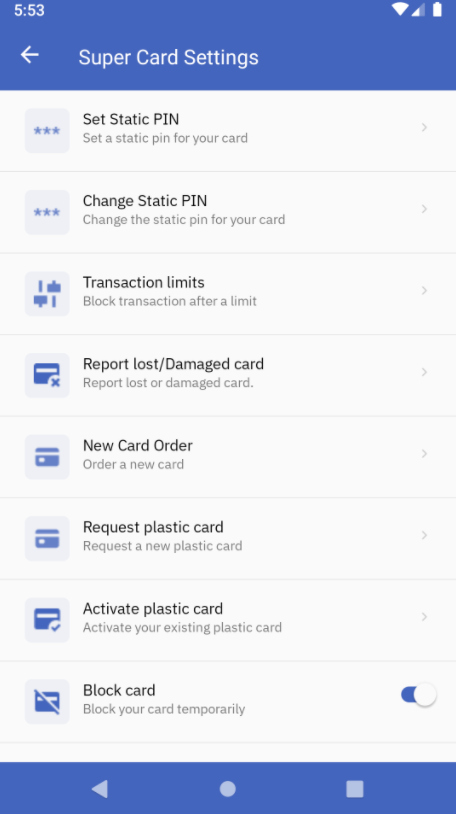
Design 2
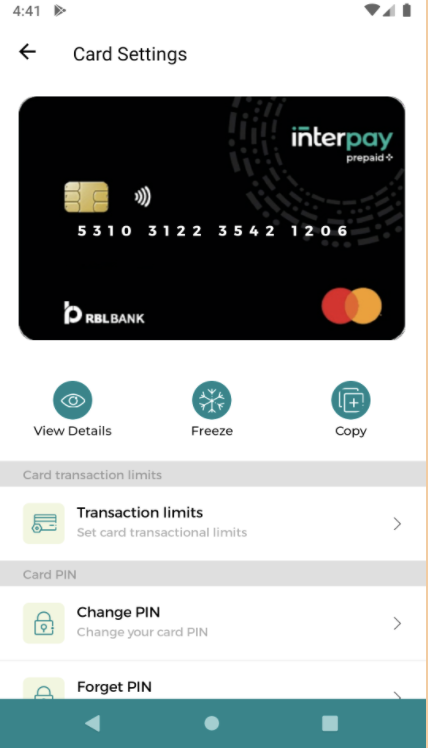
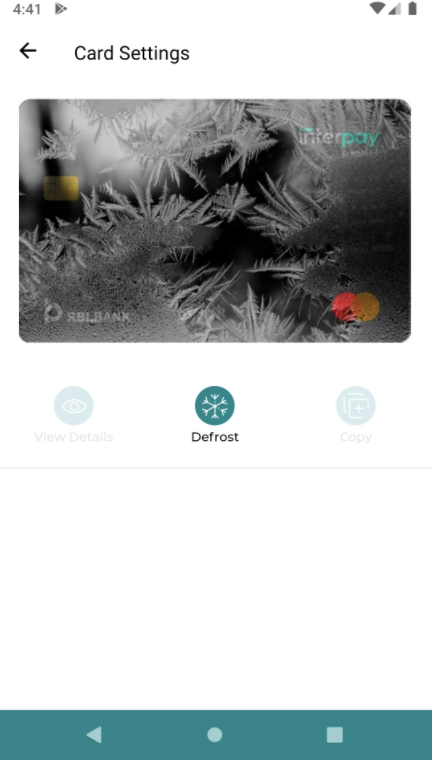
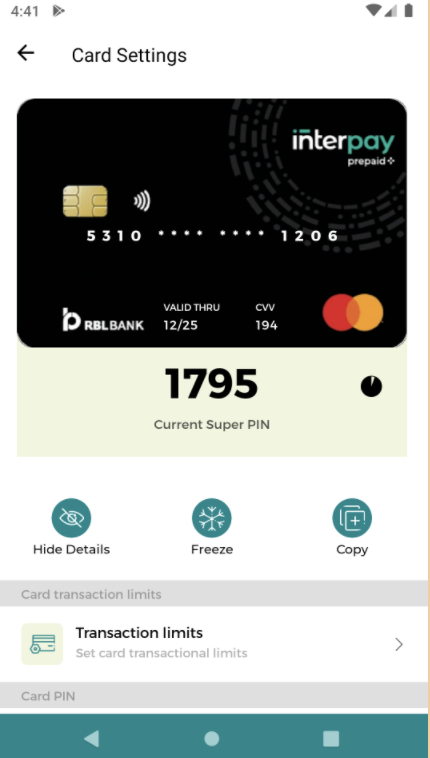
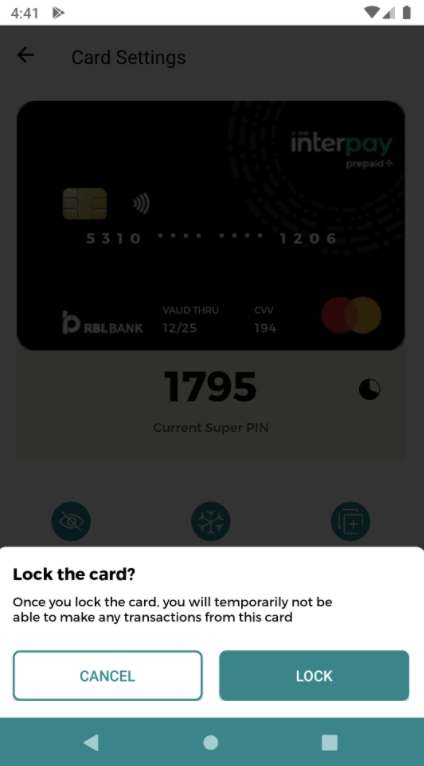